Using Vim on Mac OS - basic settings
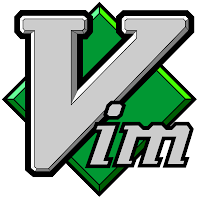
Ancient roots
Vim was released 21 years ago so most of us can argue that is is an ancient editor. An editor from an era where even Java wasn't invented (it was released in 1995). This reason alone could invalidate its usage as so many things changed since then. Well, the thing may not be that different. We are still using some kind of text editors to write source code and the problems around code writing are roughly the same: quickly edit and reformat specially formatted texts.
From the first look Vim is an editor that does not even support mouse and even deleting a line is troublesome - not to mention exiting the editor. However (given that we are willing to sacrifice couple of hours to learn the basics) Vim is a very-very capable and customizable editor. Most of the very fundamental features that helps code writing are there: autocompletion, autoformatting indents, jumping between functions, syntax highlighting and so on. It's true that default settings of the editor help to hide the interesting features, so let's have a look how to make Vim not suck.
How to make Vim not suck
To change the inconvenient defaults create a file called .vimrc under home directory and add these settings to it:
:syntax on " Highlight the keywords based on filetype
:set ruler " Display the current line number in the status bar
:set autoindent " New lines will keep the previous indentation
filetype indent on " Enable file type based indentation
filetype plugin on " Enable file type based plugins
:set ignorecase " When searching (/) will ignore the case of the input
:set smartcase " Don't ignore case when the term contains any uppercase
" :set paste " To ignore auto-indent when pasting from the clipboard
:set hlsearch " Highlight search results. To clear use :noh
:set incsearch " Search incrementally, as you type
:map <c-w> :tabclose<cr> " CTRL+W will close the current tab
:map <c-t> :tabnew<cr> " CTRL+T opens a new tab
:nnoremap <leader>s :w<cr> " \s will save the current file
:map <tab> :tabNext<cr> " Jump to next tab
:nnoremap <c-n> :set invrelativenumber<cr> " relative line #s
:set backspace=indent,eol,start " fix BS, del
:nnoremap <s-tab> :tabprevious<cr> " Jump to previous tab
:set laststatus=2 " Always display status line
autocmd filetype javascript nnoremap <leader>ff :let ln=line('.')<cr>:%!~/Documents/js-beautify/python/js-beautify -i<cr>:<c-r>=ln<cr><cr>zz:unlet ln<cr> " Run JSBeautify on the current buffer
autocmd filetype cpp nnoremap <leader>ff :let ln=line('.')<cr>:%!~/Documents/astyle/astyle --style=google<cr>:<c-r>=ln<cr><cr>zz:unlet ln<cr> " Run AStyle on the current buffer
:set expandtab " Use spaces instead of TAB char
:set tabstop=4 " 4 space= 1 tab
:set shiftwidth=4 " Indent is 4 spaces
:set noswapfile " Don't use swap/temp files
For basic code editing these settings are sufficient. However, for more serious coding it makes sense to enable code autocompletion.
Introducing neocomplcache
Vim by default supports autocompletion but it requires to press funny key combinations like <CTRL+X> - <CTRL+O> to list the suggestions (this will bring up the OmniComplete dialog). However, it can be a bit inconvenient to use, so adding a plugin that automatically opens the completion dialog on typing is much easier. There are a lot of different plugins that help with this, one of them is neocomplcache.
To install, add this line to .vimrc:
let g:neocomplcache_enable_at_startup = 1
And download the neocomplcache source from here.
Make a directory under home called .vim and copy the content of the downloaded source into it. To verify that the plugin is loaded after startup, check it using the
:scriptnames
If everything is set up properly when editing a code or text file the purple autocomplete popup should show when it finds a chunk of word that was mentioned before in this or other currently opened file.
Alternatively there is another plugin called YouCompleteMe which is a bit heavier but very popular autocomplete. It worth giving it a try.
Running external programs on current file
Vim may fail on you if you want to have fancy code formatting so in some cases the easiest solution is to rely on external code formatters (note: if you are lucky enough, someone created these as Vim plugins as well). To dump the current file to a programs standard input and replace the current file with the program's standard output, simply create a mapping for a specific file type like this:
autocmd FileType javascript nnoremap <buffer> <leader>ff :%!~/temp/js-beautify/js-beautify -t -i <cr>
When pressing \ff in a Javascript file it will invoke jsbeautifier using stdin (-i) using tab formatting (-t) and replace the content with the result. It's important that it's using the (not yet) saved buffer so if the changes look bad we don't need to save it.
To store the position before the format and restore it, use:
:let lastpos=getpos('.')<cr>:call setpos('.',lastpos)<cr>
To store the position before the format and restore it, use:
:let lastpos=getpos('.')<cr>:call setpos('.',lastpos)<cr>
Folding
It was quite a pleasant discovery when I noticed that Vim does support code folding too. It means that any other decent IDE it is able to collapse larger chunks of code (like methods and classes) so it's easier to navigate around in the code. To enable the default folding for Javascript, simply add this to .vimrc:
let javaScript_fold=1
Have a look at the cheat sheet to learn how to open/close the folds.
With Vim the learning process is pretty much never finished, we just get to a point that is enough for us. However, once in a while it worth revisiting how others use Vim to make sure we are not missing something obvious. Of course, a Vim cheat sheet can come handy too. Love it, hate it? Give it a go - there is always room to turn back!
Updates
Speed up HTML creation
It's quite typical that we need to create a complex HTML tree structure when editing a document but it can be a bit time consuming. Creating the following takes an effort:
<footer id="pageFooter">
<ul>
<li>
<a href='/contact'>Contact us</a>
</li>
<li>
<a href='/legal'>Copyright</a>
</li>
</ul>
</footer>
However, there is a very handy plugin that can generate this code using the following syntax only:
footer#pageFooter>ul>li*2>a
When pressing CTRL+Y then , (comma) it will expand to the above - of course without the textual content. Have a look, it might save you some time - Zen Coding for Vim.
In case the auto-indentation seems to be incorrect, use the Javascript indenter plugin.
In case the auto-indentation seems to be incorrect, use the Javascript indenter plugin.
Fixing HTML5 syntax highlight
Vim by default does not recognize the new HTML5 tags like footer or video. The easy fix is to add the following the the .vim/after/syntax/html.vim file:
" HTML 5 tags
syn keyword htmlTagName contained article aside audio bb canvas command datagrid
syn keyword htmlTagName contained datalist details dialog embed figure footer
syn keyword htmlTagName contained header hgroup keygen mark meter nav output
syn keyword htmlTagName contained progress time ruby rt rp section time video
" HTML 5 arguments
syn keyword htmlArg contained autofocus placeholder min max step
syn keyword htmlArg contained contenteditable contextmenu draggable hidden item
syn keyword htmlArg contained itemprop list subject spellcheck
" this doesn't work because default syntax file alredy define a 'data' attribute
syn match htmlArg "\<\(data-[\-a-zA-Z0-9_]\+\)=" contained
Comments
Post a Comment