Generate & resize different Android launcher icon sizes with a Python script
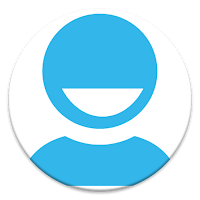
When you change your launcher icon in Android, you have to create four different sizes (96x96, 72x72, 48x48, and 36x36) for different screen sizes. The process is even more tedious when you want to have two or more different icons for different resolutions.
I've created a very simple Python script that does the resizing for you. Usually you start with the "High Resolution Application Icon" (512x512) which you create for Google Play then just simply run this script to generate the launcher icon sizes.
The script requires to have the Python PIL (imaging library) installed. On Mac OS X, follow these steps to install PIL:
Install XCode is you don't have already. Install XCode command line tools (Xcode -> Preferences -> Downloads -> Commande Line Tools -> Install) #Download & extract PIL curl -O -L http://effbot.org/downloads/Imaging-1.1.7.tar.gz tar -xzf Imaging-1.1.7.tar.gz #Build PIL cd Imaging-1.1.7 python setup.py build #Install python setup.py install --user #for all users install, use: sudo python setup.py install
When PIL is installed, you can run the following Python script:
import Image import argparse import os; res = [96,72,48,36] parser = argparse.ArgumentParser(description='Create Android launcher icons from a larger input (png output will be: 96, 72, 48, 36)') parser.add_argument('input', metavar='input.png', help='input square image file in png format') args = parser.parse_args() imageFile = args.input fileName = os.path.splitext(imageFile)[0] imgObject = Image.open(imageFile) for r in res: width = r height = r im = imgObject.resize((width, height), Image.ANTIALIAS) ext = ".png" outFile = fileName + str(r) + ext im.save(outFile) print outFile
This will create four other files in the same directory. Just rename/place them in the proper Android build directory (96: drawable-xhdpi, 72: drawable-hdpi, 48: drawable-mdpi, 36: drawable-ldpi).
Comments
Post a Comment